We just released our app. 1 user reported this issue where they subscribed, but then see this error message:
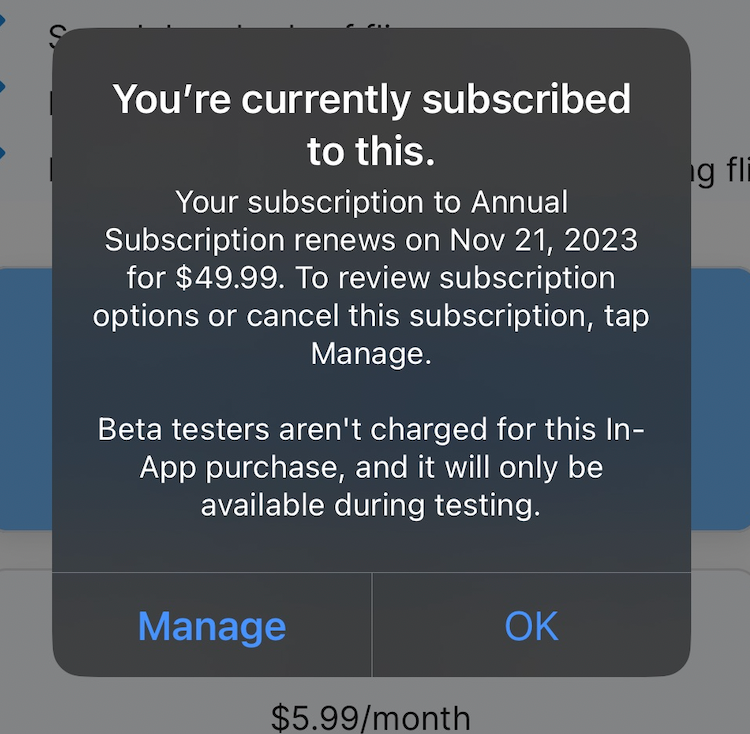
I don’t know why this happens. Here is my flow inside Expo/React Native app:
- Wrap app in providers
<PurchaseProvider setPurchasesChecked={setPurchasesChecked}> <AuthProvider> <Stack/> </AuthProvider> </PurchaseProvider>
-
Inside the
PurchaseProvider
getCustomerInfo:useEffect( function initPurchaseConfig() { const initialize = async () => { try { Purchases.setLogLevel(Purchases.LOG_LEVEL.VERBOSE); await Purchases.configure({ apiKey: APIKEY }); const info = await Purchases.getCustomerInfo(); const { isActive } = info?.entitlements.active[entitlementName] || {}; setIsActive(!!isActive); } catch (e) { setMessage(['error', (e as Error).message]); } finally { setIsReady(true); setPurchasesChecked(true); } }; initialize(); }, [setMessage, setPurchasesChecked] );
Note the
isActive
variable, which gets exported from Provider so that routes can be determined - We check if they are paywall eligible, and determine the route:
if (paywallEligible) { if (isActive) { if (!hasUser) { return router.replace('signIn'); } if ( lastSegment === 'signIn' || lastSegment === 'register' || lastSegment === 'paywall' ) { return router.replace('(tabs)'); } if (hasUser) { return; } return; } return router.replace('paywall'); } if (!hasUser) { return router.replace('signIn'); } if (lastSegment === 'signIn') { return router.replace('(tabs)'); }
In this case, if not
isActive
, they will get redirect to paywall, which lets them purchase. -
After they purchase we also set
isActive
to trueconst purchase = async (purchasePackage: PurchasesPackage) => { try { await Purchases.purchasePackage(purchasePackage); getInfo(); setIsActive(true); return router.replace(''); } catch (e) { if ( (e as PurchasesError).code === Purchases.PURCHASES_ERROR_CODE.PURCHASE_CANCELLED_ERROR ) { return; } setMessage(['error', (e as Error).message]); } };
- When I exit the app & come back, I see the “You're currently subscribed to this.” message.
it’s hard to replicate. What could this be?