When I was originally developing using the PurchasesAreCompletedBy.REVENUECAT
mode, everything worked fine.
However, since I needed to change the GoogleReplacementMode
to DEFERRED
, I had to switch to the PurchasesAreCompletedBy.MY_APP
mode.
To support this, I passed a custom purchaseLogic
to PaywallOptions
via PaywallOptions.Builder
.
But after switching to this approach, my subscription purchases started showing a “Confirm Plan” prompt, and the subscription would end up being automatically canceled.
Even after I manually called Purchases.sharedInstance.syncPurchases()
, the issue still persisted.
Is there something wrong with my setup?
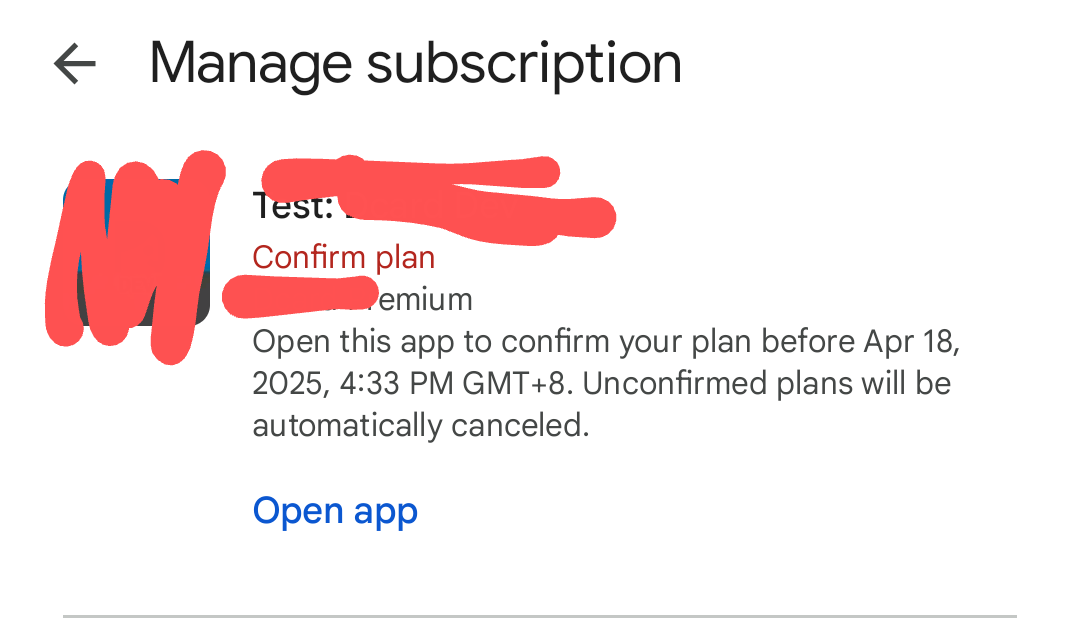
// Implementation of PurchaseLogic
override suspend fun performPurchase(
activity: Activity,
rcPackage: Package,
): PurchaseLogicResult {
onPurchaseStarted(rcPackage)
val purchaseParams = PurchaseParams.Builder(activity, rcPackage)
.googleReplacementMode(GoogleReplacementMode.WITHOUT_PRORATION)
.build()
return try {
val result = Purchases.sharedInstance.awaitPurchase(purchaseParams)
onPurchaseCompleted(result.customerInfo, result.storeTransaction)
PurchaseLogicResult.Success
} catch (e: PurchasesException) {
when (e.code) {
PurchasesErrorCode.PurchaseCancelledError -> {
onPurchaseCancelled()
PurchaseLogicResult.Cancellation
}
else -> {
onPurchaseError(e.error)
PurchaseLogicResult.Error(e.error)
}
}
}
}
Paywall(
options = PaywallOptions.Builder {
viewModel.requestToDismissPage()
}
.setOffering(null)
.setPurchaseLogic(purchaseLogic)
.build()
)